Project: Intelli
Intelli is a desktop relationship tracker used by private investigators (PI) to manage their information and leads. The PI interacts with it using a CLI, and it has a GUI created with JavaFX and GraphStream. It is written in Java, and has about 6 kLoC.
Code contributed: [Functional code] [Test code]
Locating persons: find
Finds persons whose details contain any of the given keywords.
Format: find KEYWORD [MORE_KEYWORDS]
Examples:
-
find John
Returnsjohn
andJohn Doe
. -
find Jo
Returnsjohn
andJohn Doe
. -
find Betsy Tim John
Returns any person having names or email addresses containingBetsy
,Tim
, orJohn
. -
find 92334266
Returns any person having phone number/email address/address containing92334266
. -
find Alice 92334266
Returns any person having nameAlice
AND/OR having phone number/email address/address containing92334266
.
Locating persons by name: find n/
Finds persons whose names contain any of the given keywords.
Format: find n/[KEYWORDS]
Examples:
-
find n/John
Returnsjohn
andJohn Doe
. -
find n/Jo
Returnsjohn
andJohn Doe
. -
find n/Betsy Tim John
Returns any person having namesBetsy
,Tim
, orJohn
.
You can find multiple persons with a single name search. |
Locating persons by address: find a/
Finds persons whose addresses contain any of the given keywords.
Format: find a/[KEYWORDS]
Examples:
-
find a/Serangoon
Returns any persons having addresses in Serangoon. -
find a/seRangOOn
Returns any persons having addresses in Serangoon. -
find a/Ser
Returns any persons having addresses containing the phraseSer
. -
find a/Serangoon Gardens
Returns any person having addresses containing the phraseSerangoon
AND/ORGardens
.
Locating persons by email: find e/
Finds persons whose emails contain any of the given keywords.
Format: find e/[KEYWORDS]
Examples:
-
find e/alice@example.com
ReturnsAlice
. -
find e/AliCE@ExaMPle.com
ReturnsAlice
. -
find e/@example.com
Returns any persons having email addresses containing the suffix@example.com
. -
find e/@example.com @yahoo.com
Returns any person having email addresses containing the suffix@example.com
or@yahoo.com
.
Locating persons by phone: find p/
Finds persons whose phone numbers contain any of the given keywords.
Format: find p/[KEYWORDS]
Examples:
-
find p/97734225
Returns any persons having phone numbers matching97734225
. -
find p/9773
Returns any persons having phone numbers containing the sequence9773
. -
find p/97734225 90329038
Returns any persons having phone numbers matching97734225
OR90329038
.
Locating persons by tag: find t/
Finds persons whose tags contain any of the given keywords.
Format: find t/[KEYWORDS]
Examples:
-
find t/friends
Returns any persons having tags matchingfriends
. -
find t/FriEndS
Returns any persons having tags matchingfriends
. -
find t/frIe
Returns any persons having tags containing the phrasefrie
. -
find t/friends family
Returns any persons having tags matchingfriends
AND/ORfamily
.
End of Extract ---
Justification
This offers a lot of flexibility for users who wish to find different information, and does not limit them to a simple name search. ---
Enhanced find
command
A basic find
command is given in the original codebase which allows persons to be found using their names.
In order to enhance the find
command, the find
command is extended to support searches by name/email/phone number/address/tag. This is implemented in the style of the add
command, and restricts the search to a particular aspect through parsing prefixes (/n
, /p
, /e
, /a
, /t
). Without a prefix (i.e. find
), the search is global, and returns any matches in any aspects (name/email/phone number/address/tag).
Design Considerations
Aspect: Implementation for enhanced find
command
Alternative 1 (current choice): Adapt current FindCommand
and add new predicates for each new type of search.
Pros: Easier to implement since we can reuse existing resources such as ArgumentTokenizer
used in add
command.
Cons: Implementation is less modular.
Alternative 2: Add a new subclass inheriting from FindCommand
for each new type of search.
Pros: Implementation is more modular, and developers can update code more easily.
Cons: More tedious to implement since we cannot make use of existing resources such as ArgumentTokenizer
used in add
command.
Aspect: Type of command implemented
Alternative 1 (current choice): find
command inherits from Command
instead of UndoableCommand.
Pros: Easier to implement since we do not need to keep track of previous searches.
Cons: User has to retype all search fields if user wants to repeat a particular search.
Alternative 2: find
command inherits from UndoableCommand
.
Pros: find
becomes undoable, and user can repeat a find easily by undoing the command.
Cons: It is unlikely that a user will repeat a find
multiple times in a short period of time, rendering this option somewhat unnecessary.
Aspect: User input for narrowed search field in enhanced find
command
Alternative 1 (current choice): Parse prefix in user input to narrow the search to a particular aspect (e.g. n/
).
Pros: Easier for user for learn command since it is similar to the add
command format.
Cons: Must remember exact prefix for each search field (i.e. /n
, /p
, /e
, /a
, /t
).
Alternative 2: Parse full keywords in user input to narrow the search to a particular aspect (e.g. name/
).
Pros: More intuitive for users as they can simply type what they want to look for.
Cons: User has to type longer commands.
Aspect: Scope of narrowed search
Alternative 1 (current choice): Users can only search within a single narrowed field, or must search through all possible fields (e.g. cannot search for names and emails only).
Pros: Easier to implement, parser only needs to check for a single prefix type and create corresponding predicate for the prefix type.
Cons: Fewer search choices available for users.
Alternative 2: Users can search within an arbitrary number of narrowed fields (e.g. can search for names and emails only, or search for names, emails, and addresses).
Pros: More search options available for users, more advanced search functions.
Cons: Harder to implement since the parser will have to handle more prefixes.
End of Extract ---
Editing a relationship between two persons: editRelationship
Edits a relationship between two persons in Intelli
Format: editRelationship INDEX_ONE INDEX_TWO [n/NAME] [ce/CONFIDENCE_ESTIMATE]
Examples:
-
editRelationship 1 2
-
editRelationship 1 2 n/friends
-
editRelationship 1 2 ce/3.12082
-
editRelationship 1 2 n/friends ce/3.12082
End of Extract ---
Justification
This allows users to edit relationship attributes using a single operation. This is particularly useful since the target audience is private investigators who are likely to change and update relationship information frequently after they have gathered more intelligence. ---
editRelationship
command
The editRelationship
command edits a relationship between two persons in Intelli. Using this command, users can update the names and confidence estimates of relationships existing in Intelli without having to delete and add a new relationship.
Design Considerations
Aspect: Implementation for editRelationship
command
Alternative 1 (current choice): The command is implemented internally as deleting an existing relationship and then adding a new relationship with the new name and the new confidence estimate.
Pros: Implementation does not have to access the internal state of a Relationship
, and allows for immediate updates to the graph.
Cons: Additional step involved in having to delete and then add a new relationship between the same two persons.
Alternative 2: The command directly accesses and modifies the internal state of a Relationship
.
Pros: The command can be completed in a single operation, which may be faster then deleting and adding a new relationship.
Cons: The change cannot be reflected dynamically on the graph after a successful edit.
Aspect: Type of command implemented
Alternative 1 (current choice): editRelationship
command extends UndoableCommand
instead of Command
.
Pros: Users can easily undo their changes if they accidentally update the wrong relationship attribute or have mistakes in the updates (i.e. in the new relationship name/ confidence estimate).
Cons: Additional memory needed for recording the previous stage of the address book.
Alternative 2: editRelationship
command extends Command
.
Pros: No additional memory needed for recording the previous stage of the address book.
Cons: Users can do another editRelationship
command to achieve the undo
effect.
End of Extract ---
Autocompleting commands : Tab
Completes a command by filling the command box with the first suggestion shown in the result display.
Format: INPUT
, then pressing Tab
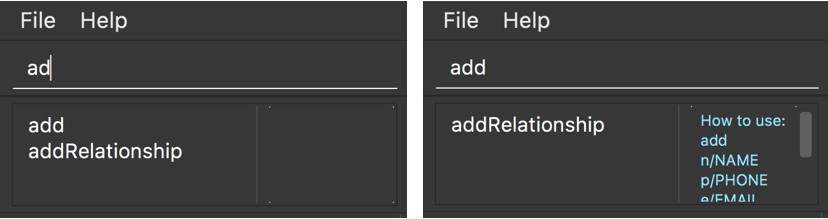
Examples:
-
Type
a
followed by Tab -
Type
add
followed by Tab
Clearing the command box : Control
Clears the command box instantaneously.
Format: Control
End of Extract ---
Justification
Certain commands are lengthy, and users who would like to enter commands quickly will find this a handy feature.
Additionally, users easily see what commands are supported by Intelli since all commands are displayed as valid suggestions if the user does not input any value in the command box.
Implementation
This has been implemented by coupling the command box and the result display. When there is a change to the user input in the command box, the suggestion displayed in the result display will change accordingly.
Enhancement Added: Command information display
External behavior
When the user types in the command box, and the input matches a command word, the corresponding usage information will be displayed in a side panel.
Justification
There are commands which require a number of parameters, and this can be a hassle for users to remember.
Implementation
This is done by adding a new display component to the main window, and binding changes to the command box to this new component.
Enhancement Added: Integrated graph display
External behavior
This ensures that the graph display is successfully integrated into the application instead of opening an additional window.
Justification
The application should be a coherent whole instead of splitting into two unsightly windows.
Implementation
This is done with the help of Java SwingNodes and JComponents. The graph is embedded in a SwingNode and intialised when the application starts up..
Enhancement Added: Enhanced graph display
Justification
This improves the user interface, making it more convenient for users to view their contacts and relationships on the graph.
Additionally, this adds to the aesthetic appeal of the application.
Other contributions
-
Tidy up the user guide